We had been using an online fax that sends faxes as a pdf attachment to email. We didn’t want to manage the email account, but instead wanted to create a script to strip the attachments and then convert them to compressed tiff format.
You’ll need python and imagemagick installed to make it all work. in python you’ll also need to install the poplib and email modules. you can install these using pip:
pip install poplib
pip install email
if you are using gmail or mail hosted off of google, you’ll need to change your security settings. First, click your account in the upper right, then choose “manage your google account”. Select security from the menu on the left, then scroll down looking for “less secure app access”. Choose Less Secure app access, and turn it on.
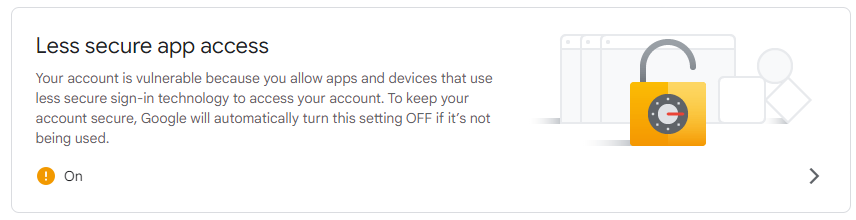
the following is the python code I had put together to get this to work.
#!/usr/bin/python3
import poplib
import email
import os
import time
from subprocess import Popen, PIPE
'''
Python script to pull pdf atachments from email
Written by: David Hedges:: dhedges at hedgesadvantage dot com
'''
savedir = "<path to save location>"
server = poplib.POP3_SSL('pop.gmail.com', '995')
server.user('<email login')
server.pass_('<email password>')
emails, tbytes = server.stat()
msg_list = server.list()
def pdf2tiff(dpath,name):
'''convert the pdf to tiff'''
newf = name.replace('.pdf', '.tif')
os.chdir(dpath)
runner = Popen(['convert -density 200x100 -compress zip -depth 1 '+name+' '+newf], shell=True, stdout=PIPE).wait()
'''fix perms'''
os.chmod(dpath+'/'+name, 0o777)
os.chmod(dpath+'/'+newf, 0o777)
'''remove pdf'''
os.remove(dpath+'/'+name)
for i in range(emails):
msg_data = server.retr(i+1)
lines = msg_data[1]
str_message = email.message_from_bytes(b'\n'.join(lines))
#print(str_message)
for part in str_message.walk():
print(part.get_content_type())
if part.get_content_type() == "application/pdf":
filename = part.get_filename()
if not(filename):
filename = "test.pdf"
#print(filename)
tvar = time.strftime("%H%M%S")
fname = filename.replace('.pdf', tvar+'.pdf')
fp = open(os.path.join(savedir, fname), 'wb')
fp.write(part.get_payload(decode=1))
fp.close
pdf2tiff(savedir, fname)
server.dele(i+1)
server.quit()
Leave a Reply